Address autocomplete fields have become a staple in web forms, improving user experience by speeding up the entry process and reducing errors. These fields are powered by address autofill APIs and address lookup APIs, which leverage vast databases to suggest addresses as users type. Implementing an address autocomplete field is easier than ever, thanks to the robust APIs available today. This guide will walk you through the steps to create an address autocomplete field, highlighting the benefits, APIs to consider, and the technical implementation.
The Importance of Address Autocomplete
Before diving into the technical details, it’s important to understand why address autocomplete fields are valuable:
1. Improved User Experience: Address autocomplete fields reduce the amount of typing required by the user, making forms quicker and easier to fill out.
2. Reduced Errors: By suggesting valid addresses, these fields help prevent common errors like typos and incorrect formatting, ensuring that the data collected is accurate.
3. Increased Efficiency: For businesses, accurate addresses mean fewer issues with delivery and correspondence, leading to better service and customer satisfaction.
Key APIs for Address Autocomplete
There are several address autofill APIs and address lookup APIs available, each with its features and advantages. Here are a few popular ones:
1. Google Places API: One of the most widely used APIs, it offers comprehensive address data and is highly reliable.
2. HERE Geocoding & Search API: Provides robust address search capabilities and is known for its accuracy and extensive database.
3. Mapbox Search API: A strong competitor with a focus on high-quality geospatial data.
4. TomTom Search API: Known for its precision, especially in Europe.
5. SmartyStreets API: Specializes in U.S. addresses, offering excellent data validation and accuracy.
Implementing Address Autocomplete: A Step-by-Step Guide
To illustrate the process of creating an address autocomplete field, we’ll use the Google Places API. The principles are similar for other APIs, but you may need to adjust some details based on the specific API you choose.
Step 1: Get Your API Key
First, you need to sign up for an API key from the service provider. For Google Places API:
1. Go to the [Google Cloud Console](https://console.cloud.google.com/).
2. Create a new project or select an existing one.
3. Navigate to the API & Services > Credentials.
4. Click “Create credentials” and select “API key”.
5. Enable the Places API for your project.
Step 2: Set Up Your HTML
Create a basic HTML file with an input field where users will type their address:
Replace `YOUR_API_KEY` with the API key you obtained from Google Cloud.
Step 3: JavaScript for Autocomplete
Create a JavaScript file named `autocomplete.js` to handle the autocomplete functionality:
function initAutocomplete() { const autocompleteInput = document.getElementById(‘autocomplete’); const autocomplete = new google.maps.places.Autocomplete(autocompleteInput, { types: [‘address’], componentRestrictions: { country: ‘us’ }, // Restrict results to the US }); autocomplete.addListener(‘place_changed’, function() { const place = autocomplete.getPlace(); if (place.geometry) { console.log(‘Address:’, place.formatted_address); console.log(‘Coordinates:’, place.geometry.location.lat(), place.geometry.location.lng()); } else { console.log(‘No details available for input:’, autocompleteInput.value); } });} document.addEventListener(‘DOMContentLoaded’, initAutocomplete);This script initializes the autocomplete functionality for the input field. The `types` option restricts suggestions to addresses, and the `componentRestrictions` option limits suggestions to a specific country (in this case, the United States).
Step 4: Enhancing User Experience
To further enhance the user experience, you can add additional features like displaying a map with the selected address, storing the selected address in a hidden field for form submission, or handling edge cases where the address is not recognized.
For example, to display a map with the selected address, you can modify the JavaScript as follows:
function initAutocomplete() { const autocompleteInput = document.getElementById(‘autocomplete’); const map = new google.maps.Map(document.getElementById(‘map’), { center: { lat: -33.8688, lng: 151.2195 }, zoom: 13, }); const marker = new google.maps.Marker({ map: map, }); const autocomplete = new google.maps.places.Autocomplete(autocompleteInput, { types: [‘address’], componentRestrictions: { country: ‘us’ }, }); autocomplete.addListener(‘place_changed’, function() { const place = autocomplete.getPlace(); if (place.geometry) { map.setCenter(place.geometry.location); marker.setPosition(place.geometry.location); marker.setVisible(true); console.log(‘Address:’, place.formatted_address); console.log(‘Coordinates:’, place.geometry.location.lat(), place.geometry.location.lng()); } else { console.log(‘No details available for input:’, autocompleteInput.value); } }); } document.addEventListener(‘DOMContentLoaded’, initAutocomplete);Add a `div` for the map in your HTML:
This enhancement provides a visual representation of the selected address, making it easier for users to verify the location.
Conclusion
Creating an address autocomplete field using an address autofill API or address lookup API significantly improves the user experience on your website. By leveraging these powerful APIs, you can provide quick, accurate, and user-friendly address suggestions that reduce errors and increase efficiency.
When selecting an API, consider factors such as data accuracy, geographical coverage, and cost. Google Places API, HERE Geocoding & Search API, Mapbox Search API, TomTom Search API, and SmartyStreets API are all excellent choices depending on your specific needs.
The implementation process involves obtaining an API key, setting up your HTML, writing JavaScript to handle the autocomplete functionality, and enhancing the user experience with additional features. By following these steps, you can easily integrate an address autocomplete field into your web forms, providing a seamless and efficient experience for your users.
In a digital world where user experience is paramount, the ease and accuracy provided by address autofill and address lookup APIs are invaluable. Embrace these tools to enhance your forms, reduce errors, and ensure your data is as accurate as possible.
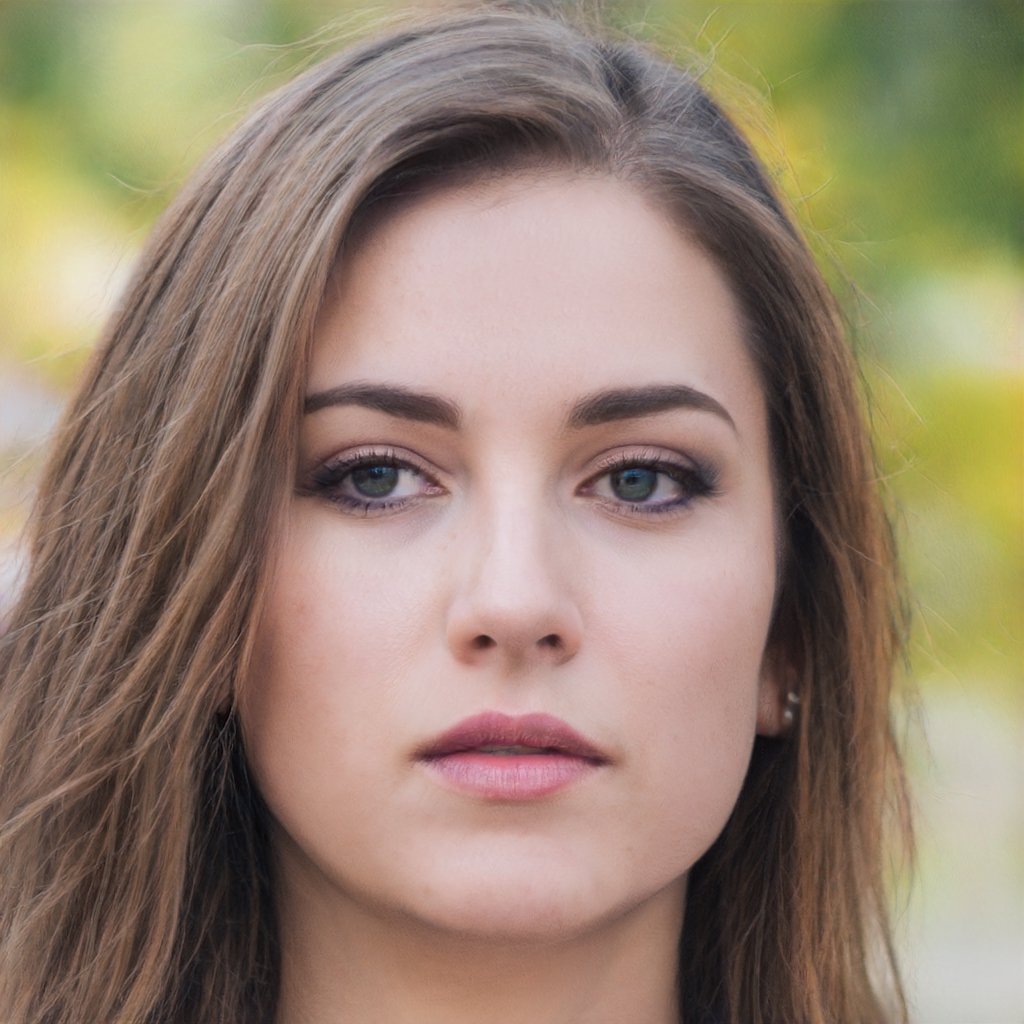
Ruby Stauffer is a prominent technology blogger known for her insightful analysis and in-depth reviews of the latest tech trends and gadgets. Her blog has become a go-to resource for tech enthusiasts seeking reliable information and expert opinions on the ever-evolving world of technology.